Sealed class and Partial class in C#

What is Sealed class?
Sealed classes are used to restrict the inheritance feature of object oriented programming. It cannot be inherited in another class. The variable & methods in sealed class can be accessed in main().
Example of Sealed class:
using System; using System.Collections; namespace Demo11 { sealed class Customer { public int cid; public String cname; public void getdetails() { Console.WriteLine(cid + " " + cname); } } class Demo { public static void Main() { Customer obj = new Customer(); obj.cid = 101; obj.cname = "Kamlesh"; obj.getdetails(); Console.Read(); } } }
Output:

What is Partial class?
Partial class means incomplete class. It is used to place the code in different classes. Multiple partial classes will have the same name & will be accessed with one object name. Partial class is useful when multiple programmers are writing code for one class.
All the partial class definitions must be in the same assembly and namespace. All the parts of partial class must have the same accessibility like public or private, etc.
Example of Partial class:
using System; using System.Collections; using Demo11; namespace Demo11 { partial class Product { public int pid; public String pname; } partial class Product { public void getdetails() { Console.WriteLine(pid+" "+pname); } } class Demo { public static void Main() { Product obj = new Product(); obj.pid = 1; obj.pname = "Microsoft"; obj.getdetails(); Console.Read(); } } }
Output:
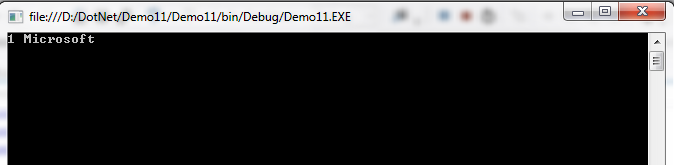
In this way, we learned sealed class and partial class in C# with examples which will help beginners to understand sealed class and partial class.
You may also be interested in...
- Slack Integration with C#
- Xamarin Forms: Getting Started
- Part 1: Introduction to C#
- Part 2: Arrays and Function in C#
- Part 3: OOPs Concepts in C#
- Part 4: Constructor/Destructor in C#
- Part 5: Abstract class in C#
- Part 6: Indexer,Delegates, Anonymous Method, Lambda expression in C#
- Part 7: Collections in C#
- Part 8: Generics in C#
- Part 9: Attributes in C#
- Part 10: Sealed/Partial class in C#
- Part 11: MultiThreading in C#
Happy Coding!
Discuss about post