Object Oriented Programming Concepts in C#

Introduction:
Object Oriented Programming means set of rules and rules are converted to concepts or features. Object Oriented Programming is used for code suppretion. The code can be suppreted by creating classes and Object Oriented Programming is used to perform multitask. Multitask means getting more than one result at a time. In Object Oriented Programming, class is used to create new datatype or user defined datatype. Class contains variables and methods. Variables are used to store values and methods are used to Manipulate the values. By using a class, object can be created. Object contains state and behaviour. State is used to tell what the object is and behaviour is used to tell what the object can do.
Note:
If class is created with variables and methods then memory will not be preserved for them. Memory will be preserved only when object is created. When object is created using new operator then object will be stored in a heap memory. When object is stored in heap memory then it will contain the reference or memory address of the class. By using object, variables and methods in the class can be accessed with the object name by using dot(.) operator. This operator is called member access operator.
Access Specifiers in C#:
- Public
- Private
- Protected
- Internal
- Protected internal
The variables & methods declared as public can be accessed in other classes and can be accessed in main().
If the variables & methods declared as private then they can be accessed within the class. They cannot be accessed in other classes & cannot be accessed even in main().
For the vriables & methods if access specifier is not given then default access specifier is private.
Object Oriented Programming Concepts or Features:
1)Encapsulation:
Wrapping of variables & methods as a unit or else as a block is known as encapsulation. If a class is created with variables & methods then it is considered as a encapsulation.
2)Inheritance:
Inheritance means reusability or reusing existing code. The variables and methods existing in one class can be used in other classes.
* Types Of Inheritance:
- Single inheritance
- Multiple inheritance
- Multilevel inheritance
- Hierarchical inheritance
- Hybrid inheritance
In C#, multiple inheritance is not possible with classes. Instead of classes, interfaces are used.
3)Polymorphism:
Polymorphism means, "One name, multiple results". Ploymorphism is of two types: Compile time polymorphism & Runtime polymorphism. Compile time polymorphism can be called as static polymorphism or 'Early binding'. Runtime polymorphism is called dynamic polymorphism or 'Late binding'. Function overloading and operator overloading are the examples of compile time polymorphism. Function overriding or virtual function are the examples of runtime polymorphism.
i)Method overloading:
A class can contain multiple functions with the same name but there should be difference in number of arguments, order of arguments or difference in data types. It is called as compile time polymorphism i.e based on the given arguments or values while calling the function, compiler will decide at compilation time that which method in the class should execute.
Programs:
1) Example of method overloading:
using System; namespace Demo { class Program { public void Sum(int a, int b) { Console.WriteLine(a+b); } public void Sum(int a, int b, int c) { Console.WriteLine(a + b + c); } static void Main(string[] args) { Program obj = new Program(); obj.Sum(10, 20); obj.Sum(10, 20, 30); Console.Read(); } } }
Output:

2) Example of method overloading with different datatype as arguments:
using System; namespace Demo { class Program { public void getVal(int a, string s) { Console.WriteLine(a + " " + s); } public void getVal(string s, int a) { Console.WriteLine(s + " " + a); } } class Demo { static void Main(string[] args) { Program obj = new Program(); obj.getVal(10, "Kamlesh"); obj.getVal("Dotnetwisdom.com", 1); Console.Read(); } } }
Output:

Inheritance:
In inheritance, base class and derived class is used. Base class can be called as independent class or parent class. Derived class means, creating a class by using another class. This class can be called as dependent class or child class. If one base class is used in a another derived class then it is called single inheritance. If two interfaces are used in one class then it is called multiple inheritance. If derived class is used in another class then it is called multilevel inheritance.
Programs:
1) Using base class in derived class:
using System; namespace Demo { class clsEmp { public int eno; public string ename; } class clsMgr: clsEmp { public int hra; public void showDetails() { Console.WriteLine(eno+"\n"+ename+"\n"+hra); } } class Demo { static void Main(string[] args) { clsMgr obj = new clsMgr(); Console.WriteLine("Enter eno, ename and hra"); obj.eno = int.Parse(Console.ReadLine()); obj.ename = Console.ReadLine(); obj.hra = int.Parse(Console.ReadLine()); obj.showDetails(); Console.Read(); } } }
Output:
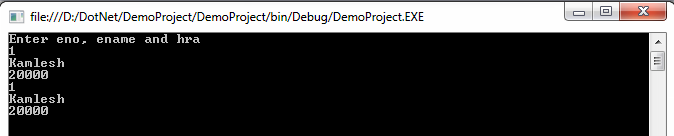
Note:
Protected - It is an access specifier. The variables and methods declared as protected can be used only in derived class. They cannot be accessed in other classes and cannot be accessed even in main().
2) Using base class method in derived class:
using System; namespace Demo { class abc { public int a,b; public int ab() { return a + b; } } class xyz: abc { public int c; public void getValues() { Console.WriteLine(ab()+ c); // Calling the function ab(). } } class Demo { static void Main(string[] args) { xyz obj = new xyz(); obj.a = 5; obj.b = 10; obj.c = 20; obj.getValues(); Console.Read(); } } }
Output:

3) Using one base class in two derived classes:
using System; namespace Demo { class abc { public int a,b; } class xyz: abc { public void Add() { Console.WriteLine("Addition is:"+(a+b)); } } class pqr : abc { public void Multiply() { Console.WriteLine("Multiplication is:"+(a * b)); } } class Demo { static void Main(string[] args) { xyz obj = new xyz(); obj.a = 5; obj.b = 10; obj.Add(); pqr obj1 = new pqr(); obj1.a = 20; obj1.b = 5; obj1.Multiply(); Console.Read(); } } }
Output:

ii) Method overriding:
Methods in base class and derived class having the same signature, then method in derived class will overrides the method in base class. Same signature means, method name, number of arguments and order of arguments and datatypes will be same. Method overriding will work as runtime polymorphism i.e.according to the method address, compiler will decide runtime that which method in the class should execute.
4) Example of method overriding:
using System; namespace Demo { class clsEmp { public virtual void getdetails(int eno, string ename) { Console.WriteLine(eno + " " + ename); } } class clsdept : clsEmp { public override void getdetails(int dno, string dname) { base.getdetails(101, "Kamlesh"); Console.WriteLine(dno + " " + dname); } } class Demo { static void Main(string[] args) { clsdept obj = new clsdept(); obj.getdetails(20, "Developer"); Console.Read(); } } }
Output:

Note:
Base class method should contain virtual so that other functions can override base class function. Derived class method will contain override, so that it can override base class method. 'base' is a predefined object created for base class. To access base class methods ,it is used.
5) Using derived class in another class:
using System; namespace Demo { class abc { public int a; } class xyz:abc { public int b; } class pqr:xyz { public int c; public void getResult() { Console.WriteLine("Addition is:"+(a + b + c)); } } class Demo { static void Main(string[] args) { pqr obj = new pqr(); obj.a = 5; obj.b = 10; obj.c = 15; obj.getResult(); Console.Read(); } } }
Output:
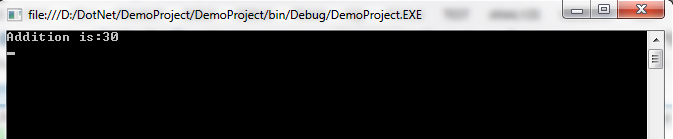
Interface:
It is similar to a class but instance or object cannot be created with the interface. Interface will contain abstract methods (method without body or statements). The abstract method given in interface will be implemented or created in a class. That class is called implementation class. Interface is used to work with multiple inheritance and used to provide security for the methods in the class.
Programs:
1) Using one interface in a class:
using System; namespace Demo { interface IEMP { void getempdetails(int eno,string ename); } class clsEmp:IEMP { void IEMP.getempdetails(int eno,string ename) { Console.WriteLine(eno+" "+ename); } } class Demo { static void Main(string[] args) { IEMP obj; //declaration obj=new clsEmp(); obj.getempdetails(100,"Kamlesh"); Console.Read(); } } }
Output:
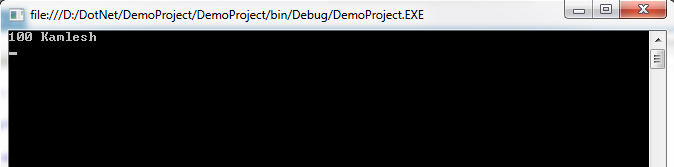
Note:
In Interface, access specifier will not be given. Default access specifier is public.
2) Using two interfaces in one class:
using System; namespace Demo { interface IEMP { void getEmpDetails(int eno,string ename); } interface iDept { void getDeptDetails(int dno, string dname); } class clsEmp:IEMP, iDept { void IEMP.getEmpDetails(int eno, string ename) { Console.WriteLine(eno+" "+ename); } void iDept.getDeptDetails(int dno, string dname) { Console.WriteLine(dno + " " + dname); } } class Demo { static void Main(string[] args) { IEMP obj1; //declaration obj1=new clsEmp(); obj1.getEmpDetails(101, "Kamlesh"); iDept obj2; obj2 = new clsEmp(); obj2.getDeptDetails(10, "Software"); Console.Read(); } } }
Output:

3) Using interface in another interface:
using System; namespace Demo { interface IEMP { void getEmpDetails(int eno,string ename); } interface iDept:IEMP { void getDeptDetails(int dno, string dname); } class clsEmp:iDept { void IEMP.getEmpDetails(int eno, string ename) { Console.WriteLine(eno+" "+ename); } void iDept.getDeptDetails(int dno, string dname) { Console.WriteLine(dno + " " + dname); } } class Demo { static void Main(string[] args) { iDept obj; //declaration obj=new clsEmp(); obj.getEmpDetails(101, "Kamlesh"); obj.getDeptDetails(20, "Software"); Console.Read(); } } }
Output:

4) Using one interface in two classes:
using System; namespace Demo { interface inter1 { void getResult(int a,int b); } class MyClass1:inter1 { void inter1.getResult(int a, int b) { Console.WriteLine("Sum is :"+(a+b)); } } class Myclass2: inter1 { void inter1.getResult(int a, int b) { Console.WriteLine("Difference is:" + (a - b)); } } class Demo { static void Main(string[] args) { inter1 obj; obj = new MyClass1(); // Initialization obj.getResult(10, 20); obj = new Myclass2(); // Re-initialization obj.getResult(50, 10); Console.Read(); } } }
Output:

Static:
If you want to use one particular value in the entire program or else in entire application then the value should be stored in the static variable. It is used to preserve memory for the variables and methods at the compilation time. If the class contains static variables and static methods then variables and methods can be accessed with class name.
Programs:
1) Example of static variables and methods:
using System; namespace Demo { class clsEmp { public static int eno; public static string ename; public static void getempdetails() { Console.WriteLine(eno+" "+ename); } public int dno; //Non static variable public string dname; public void getdeptdetails() { Console.WriteLine(dno+" "+dname); } } class Demo { static void Main(string[] args) { clsEmp.eno=222; clsEmp.ename="Kamlesh"; clsEmp.getempdetails(); clsEmp obj=new clsEmp(); obj.dno=30; obj.dname="Software"; obj.getdeptdetails(); Console.Read(); } } }
Output:
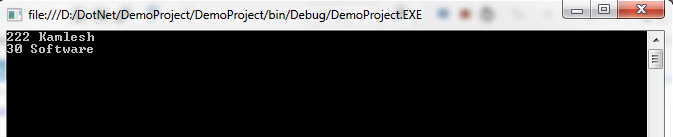
2) Example of static variables and class:
using System; namespace Demo { static class clsinfo { public static int a; public static void getA() { Console.WriteLine(a); } } class Demo { static void Main(string[] args) { clsinfo.a = 40; clsinfo.getA(); Console.Read(); } } }
Output:

In this way, we have learned Object Oriented Programming concepts with examples in this article. I hope this will help beginners to understand OOPs concepts.
You may also be interested in...
- Slack Integration with C#
- Xamarin Forms: Getting Started
- Part 1: Introduction to C#
- Part 2: Arrays and Function in C#
- Part 3: OOPs Concepts in C#
- Part 4: Constructor/Destructor in C#
- Part 5: Abstract class in C#
- Part 6: Indexer,Delegates, Anonymous Method, Lambda expression in C#
- Part 7: Collections in C#
- Part 8: Generics in C#
- Part 9: Attributes in C#
- Part 10: Sealed/Partial class in C#
- Part 11: MultiThreading in C#
Happy Coding!
Discuss about post