MultiThreading in C#

Introduction:
Thread means it is a block of code will perform a specific task when it is executed. By using multithreading, different parts of the program or different parts of an application can be executed at a time. This leads to multitask. Multitask means getting more than one result at a time. In C#, multiple methods can be created and can be executed at a time or concurrently using multithreading. To work with multithreading System.Threading namespace should be used. This namespace contains thread class. By using this class, child thread can be created and can be executed (child thread means methods).
Methods in Thread Class:
1. Start():
It is used to start the execution of the thread.
2. Sleep():
It is used to stop the execution of the thread for the given intervval of time. The interval will be in milli seconds.
3. Join():
It will not allow concurrent execution of threads. If one thread execution is completed then another thread execution will start.
4. Suspend():
It is used to stop the execution of the thread for the given interval. And again thread will start by using resume method.
Properties in Thread class:
1. Name:
It is used to assign or to access the thread name.
2. IsAlive:
It is used to specify whether the thread is alive or dead.
3. Priority:
It is used to specify which thread should execute first.
Main() is considered as main thread. When program execution is started, main thread will start first and will make the child threads to start and to finish. And Main thread execution also will be completed then program will end. Child threads will be controlled or managed by the main thread.
Examples:
1) Program to execute two methods at a time or concurrently:
using System; using System.Threading; namespace Demo { class Program { Thread T1, T2; public Program() // Creating constructor { T1 = new Thread(new ThreadStart(Fun1)); T2 = new Thread(new ThreadStart(Fun2)); T1.Start(); T1.Priority = ThreadPriority.Highest; T2.Start(); } public void Fun1() { for (int a = 1; a <= 5; a++) { Console.WriteLine("Fun1..."+a); Thread.Sleep(1000); } } public void Fun2() { for (int b = 5; b >= 1; b--) { Console.WriteLine("Fun2..." + b); Thread.Sleep(1000); } } } class demo { public static void Main() { new Program(); // Calling constructor Console.Read(); } } }
Output:
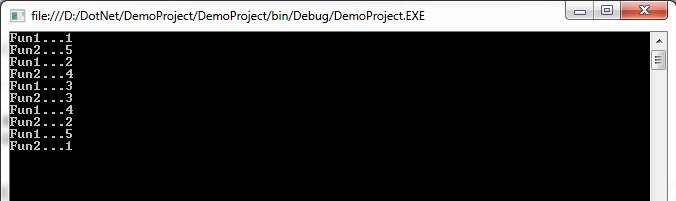
Note:
ThreadStart is the predefined delegate used to copy the reference of the method in thread variable. T1 contains memory address of Fun1 and T2 contains memory address of Fun2.
ThreadPriority is the enum or enumeration and highest is the element or value. It is used to specify which thread should start first.
2) Example using IsAlive and Join():
using System; using System.Threading; namespace Demo { class Program { Thread T1, T2; public Program() // Creating constructor { T1 = new Thread(new ThreadStart(Fun1)); T2 = new Thread(new ThreadStart(Fun2)); T1.Start(); Console.WriteLine(T1.IsAlive); T1.Join(); Console.WriteLine(T1.IsAlive); T2.Start(); } public void Fun1() { for (int a = 1; a <= 5; a++) { Console.WriteLine("Fun1..."+a); Thread.Sleep(1000); } } public void Fun2() { for (int b = 5; b >= 1; b--) { Console.WriteLine("Fun2..." + b); Thread.Sleep(1000); } } } class demo { public static void Main() { new Program(); // Calling constructor Console.Read(); } } }
Output:
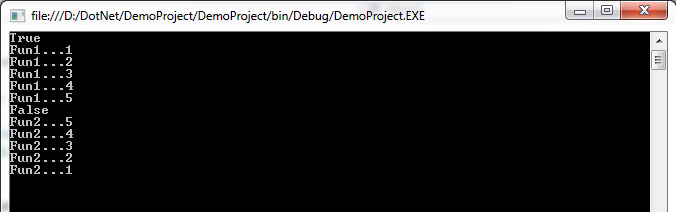
Thread Synchronization:
When multiple threads works on one object at a time or concurrently then it is called asynchronization. With asynchronization, the threads may not get the required data from the object. Synchronization means multiple threads will work on one object one after another so that required data can be accessed from the object. Synchronization is implemented by using Lock(). When any thread is using the object, it will lock the object so that it cannot be used by another threadat the same time.
Example of Thread Synchronization:
using System; using System.Threading; namespace Demo { class Temp { public void display(string s) { Console.Write("[Hello :"); Thread.Sleep(1000); Console.WriteLine(s + "]"); } } class Program { Temp obj; Thread T1, T2; public void Test() { obj = new Temp(); T1 = new Thread(new ThreadStart(Fun1)); T2 = new Thread(new ThreadStart(Fun2)); T1.Start(); T2.Start(); } public void Fun1() { lock (obj) { obj.display("Kamlesh"); } } public void Fun2() { lock (obj) { obj.display("Bhor"); } } } class demo { public static void Main() { new Program().Test(); // Default constructor Console.Read(); } } }
Output:

In this way, we learned MultiThreading in C# with examples which will help beginners to understand what is multithreading and how to implement it.
You may also be interested in...
- Slack Integration with C#
- Xamarin Forms: Getting Started
- Part 1: Introduction to C#
- Part 2: Arrays and Function in C#
- Part 3: OOPs Concepts in C#
- Part 4: Constructor/Destructor in C#
- Part 5: Abstract class in C#
- Part 6: Indexer,Delegates, Anonymous Method, Lambda expression in C#
- Part 7: Collections in C#
- Part 8: Generics in C#
- Part 9: Attributes in C#
- Part 10: Sealed/Partial class in C#
- Part 11: MultiThreading in C#
Happy Coding!
Discuss about post