Indexer, Delegates, Anonymous Method, Lambda Expression, Safe & Unsafe code in C#

Indexer:
It is used to store multiple values in one object. It can be called as array on object. If class is created with indexer and when object is created for the class then multiple values can be stored. It contains get and set block. Get block is used to access the value and set block is used to assign the value. Indexer can also be called as "smart array".
Important points about Indexer:
- Indexers are always created with this keyword.
- Indexers are implemented through get and set accessors for the [ ] operator.
- ref and out parameter modifiers are not permitted in indexer.
- Indexers are used on group of elements.
- Indexers are accessed using indexes where as properties are accessed by names.
- Indexer can be overloaded.
Examples:
1) Example of Indexer having integer values:
using System; namespace Demo11 { class myclass1 { private int[] a = new int[3]; public int this[int i] { get { return a[i]; } set { a[i] = value; } } class Test { static void Main(string[] args) { myclass1 obj = new myclass1(); obj[0] = 5; obj[1] = 10; obj[2] = 15; Console.WriteLine("{0},{1},{2}", obj[0], obj[1], obj[2]); Console.ReadLine(); } } } }
Output:

2) Example of Indexer having string values:
using System; namespace Demo11 { class myclass1 { private string[] s = new string[3]; public string this[int i] { get { return s[i]; } set { s[i] = value; } } class Test { static void Main(string[] args) { myclass1 obj = new myclass1(); obj[0] = "VC#"; obj[1] = "ASP.net"; obj[2] = "SQL Server"; Console.WriteLine("{0}\n{1}\n{2}\n", obj[0], obj[1], obj[2]); Console.ReadLine(); } } } }
Output:

Delegates:
Delegate is used to copy the reference or address of the method in another variable. It is used to execute methods on events. In windows application, controls will have events. Events are user actions. They are click, double click, key press etc. Delegate is a type or class used to store memory location of the method. Delegate is similar to function pointer in 'c' language.
Examples:
1) Create a delegate which can be used on a method with two arguments:
using System; namespace Demo11 { public delegate void mydel(int a,int b); class clsInfo { public void display(int a,int b) { { Console.WriteLine(a+b); } } class Test { static void Main(string[] args) { clsInfo obj=new clsInfo(); mydel d1=new mydel(obj.display); d1(5,10); // invoke or execute Console.ReadLine(); } } } }
Output:

Note:
To copy the reference of the method in delegate variable then the delegate signature and method signature should be same i.e number of arguments, order of arguments, datatypes should be same. In the given example delegate variable d1 will contain the memory address of the display method.
2) Create the delegate which can be used on the method containing one argument & return type string:
using System; namespace Demo11 { public delegate string mydel(int a); class clsInfo { public string fun1(int a) { { if (a > 0) return "positive"; else return "negative"; } } public string fun2(int a) { if (a > 0) return "+"; else return "-"; } } class Test { static void Main(string[] args) { clsInfo obj=new clsInfo(); mydel d1=new mydel(obj.fun1); Console.WriteLine(d1(-10)); mydel d2=new mydel(obj.fun2); Console.WriteLine(d2(-5)); Console.ReadLine(); } } }
Output:

3) Create a delegate which can be used on a method with number of arguments:
using System; namespace Demo11 { public delegate void mydelegate(); class clsInfo { public void printmsg() { Console.WriteLine("VC#"); } } class Test { static void Main(string[] args) { clsInfo obj=new clsInfo(); mydelegate d1=new mydelegate(obj.printmsg); d1(); Console.ReadLine(); } } }
Output:

Anonymous Method:
Using anonymous method set of statements can be executed while creating variable for the delegate. With this creating class containing method & creating object for the class is not required.
Examples:
1) Example of Anonymous Method:
using System; namespace Demo11 { public delegate void mydel(); class demo { static void Main(string[] args) { mydel d1=delegate()// Anonymous method { Console.WriteLine("VC#"); }; d1(); Console.Read(); } } }
Output:

2) Create a delegate which can be used on anonymous method, with two arguments:
using System; namespace Demo11 { public delegate void mydel(int a,int b); class demo { static void Main(string[] args) { mydel d1=delegate(int a,int b)// Anonymous method { Console.WriteLine("a+b"); }; d1(10, 20); Console.Read(); } } }
Output:
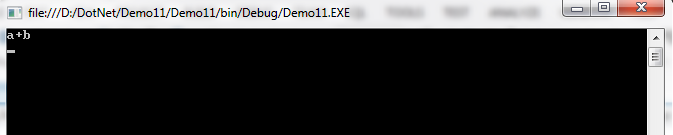
Lambda Expression:
While working with anonymous method, datatypes, braces, return keyword should be used. With Lambda expression without using all this the result will be displayed.
Example of Lambda Expression:
using System; namespace Demo11 { public delegate int mydel(int a,int b); class demo { static void Main(string[] args) { mydel d1=delegate(int a,int b)// Anonymous method { return a + b; }; Console.WriteLine(d1(5, 10)); mydel d2 = (a, b) => a + b; //Lambda expression Console.WriteLine(d2(10, 12)); Console.Read(); } } }
Output:

Multicast Delegate:
It is used to execute multiple methods in delegate variables one by one or one after another. The delegate signature & multiple method signature should be same. To copy the method address in delegate variable "+=" is used. To remove method address from delegate variable "-=" is used.
Safe & Unsafe Code:
Both will works with memory location or memory address. Safe code means working with ref & delegates. Unsafe code means working with pointers. Pointers will store or will hold the memory address of the value. In pointers, two symbols are used. i.e (*,&). '*' is used to get the value from memory address. '&' is used to get memory address of the value.
Example of Safe & Unsafe code:
using System; namespace Demo11 { class demo { unsafe static void getval(int* p) { Console.WriteLine(*p); } unsafe static void Main() { int a = 5; getval(&a); } } }
Output:
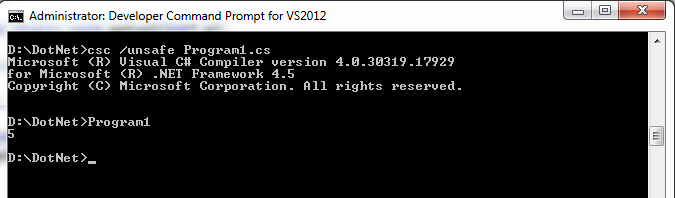
Type this program in notepad & save the file as D:\DotNet>Progeam1.cs. click on program-->visual studio 2012-->Visual Studio Tools-->developer command prompt of VS.net 2012
D:\DotNet>
To compile:
D:\DotNet>csc /unsafe Program1.cs
To execute:
D:\DotNet>Program1
Note:
In the given example, call by reference is used. i.e while calling or executing the function the reference of the value or memory address of the value will be sent to function creation.
In this way, we have learned Indexer, Delegates, Anonymous Method, Lambda Expression, Safe & Unsafe code in C# with examples in this article. I hope this will help beginners to understand what is Indexer, Delegates, Anonymous Method, Lambda Expression, Safe & Unsafe code and how to implement it.
You may also be interested in...
- Slack Integration with C#
- Xamarin Forms: Getting Started
- Part 1: Introduction to C#
- Part 2: Arrays and Function in C#
- Part 3: OOPs Concepts in C#
- Part 4: Constructor/Destructor in C#
- Part 5: Abstract class in C#
- Part 6: Indexer,Delegates, Anonymous Method, Lambda expression in C#
- Part 7: Collections in C#
- Part 8: Generics in C#
- Part 9: Attributes in C#
- Part 10: Sealed/Partial class in C#
- Part 11: MultiThreading in C#
Happy Coding!
Discuss about post