Collections in C#

What is Collection?
Collection is a class used to store set of values. If class contains indexer then it is called as collection. The predefined collectiions are existed in System.Collections namespace. To overcome the drawbacks of array, Collections are used.
Difference Between Arrays and Collections:
Arrays | Collections |
---|---|
It has fixed size | It has no fixed size |
Used to store similar types data | Used to store composite or mixed data(int, char & float values) |
After storing the values, adding new value, editing & deleting old values is very difficult | Collection contains set of methods. By using them adding, editing & deleting of values can be done easily |
If you want to store values & if you want to access them then arrays are best. They will work fast & are very difficult. If you want to store the values & after that if you want to manipulate the values then collections are best.
1. ArrayList:
It is the predefined collection used to store composite or mixed data. when multiple values are stored in collection they will be stored based on index value. The index value starts with zero(0).
Examples:
1. Example of ArrayList:
using System.Collections; using System; namespace Demo11 { class demo { public static void Main(string[] args) { ArrayList List = new ArrayList(); List.Add(101); List.Add("Kamlesh"); List.Add(50000.99); List.Insert(2, "Manager"); foreach (object s in List) { Console.WriteLine(s); } Console.WriteLine("count is:" + List.Count); Console.Read(); } } }
Output:
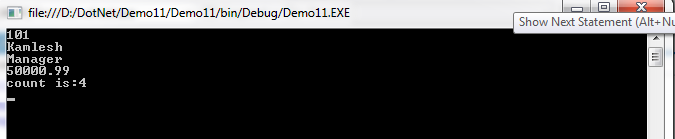
Methods in ArrayList collection:
- Add() : It is used to add value to the collection.
- AddRange() : It is used to add set of values to the collection.
- Insert() : It is used to add new value in between existing values.
- Remove() : It is used to delete specified values from collection.
- RemoveAt() : It is used to delete value from collection based on the given index value.
- Clear() : It is used to delete all the values from collection.
- IndexOf() : It is used to get Index value of the specified value in the collection.
- Sort() : It is used to show values in ascending order.
Count:
It is the property in ArrayList collection used to get count of values.
2. Example of ArrayList using Property:
using System; using System.Collections; namespace Demo11 { class Employee { private int eno; private string ename; public Employee(int eno, string ename) //Creating Constructor { peno = eno; // Property pename = ename; } public int peno // Property name { get { return eno; } set { eno = value; } } public string pename // Property name { get { return ename; } set { ename = value; } } } class Demo { public static void Main(string[] args) { ArrayList list = new ArrayList(); list.Add(new Employee(1001, "Kamlesh")); // Calling Constructor list.Add(new Employee(1002, "Dotnetwisdom.com")); foreach (Employee e in list) { Console.WriteLine(e.peno + " " + e.pename); } Console.Read(); } } }
Output:

2. SortedList:
It is used to store values in key/value pair. And the values will be sorted based on the given keys.
Example of SortedList:
using System; using System.Collections; namespace Demo11 { class Demo { public static void Main(string[] args) { SortedList sl = new SortedList(); sl.Add("a","Sunday"); sl.Add("b", "Monday"); sl.Add("c", "Tuesday"); sl.Add("d", "Wednesday"); sl.Add("e", "Thursday"); sl.Add("f", "Friday"); sl.Add("g", "Saturday"); for (int i = 0; i < sl.Count; i++) { Console.WriteLine(sl.GetKey(i) + " " + sl.GetByIndex(i)); } Console.Read(); } } }
Output:
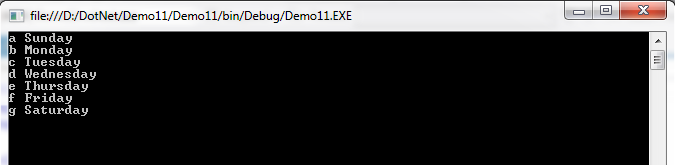
Note:
SortedList collection contains Add() method. By using that method key & value can be given. Count Property in SortedList collection is used to get count of values. GetKey() is used to get key from the collection. GetByIndex() is used to get value from the given index.
3. HashTable:
This collection will store values in key/value pair and for every key, hash code or hash value is generated. It is used to uniquely identify each value in the collection.
Example of HashTable:
using System; using System.Collections; namespace Demo11 { class Demo { public static void Main(string[] args) { Hashtable ht = new Hashtable(); ht.Add(1, "VC#"); ht.Add(2, "ASP.Net"); ht.Add(3, "Sql Server"); ht.Add(4, "MS-bi"); foreach (DictionaryEntry de in ht) { Console.WriteLine(de.GetHashCode()); Console.WriteLine(de.Key + " " + de.Value); } Console.Read(); } } }
Output:
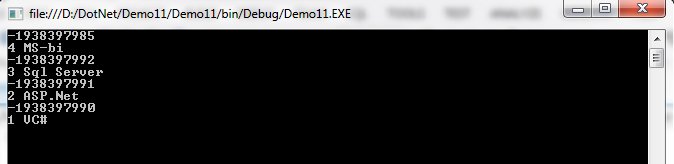
Note:
DictionaryEntry is the predefined structure used to access values from HashTable collection. GetHashCode() is used to get hash code for the given key. Key & Value properties in DictionaryEntry is used to access key and value from collection.
4. Stack:
It will store value in 'First In Last Out' order.
Example of Stack:
using System; using System.Collections; namespace Demo11 { class Demo { public static void Main(string[] args) { Stack s = new Stack(); s.Push(10); s.Push(20); s.Push(30); foreach (int a in s) { Console.WriteLine(a); } Console.Read(); } } }
Output:
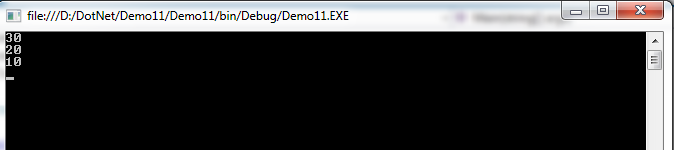
Note:
Push() is used to send values to the stack & Pop() is used to delete values from stack collection.
5. Queue:
It will store values in 'First In First Out' order.
1) Example of Queue:
using System; using System.Collections; namespace Demo11 { class Demo { public static void Main(string[] args) { Queue q = new Queue(); q.Enqueue(10); q.Enqueue(20); q.Enqueue(30); foreach (int a in q) { Console.WriteLine(a); } Console.Read(); } } }
Output:
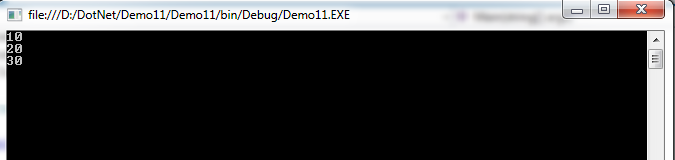
Note:
Enqueue() is used to send the values to the collection.
Dequeue() is used to delete values from the collection.
In this way, we learned Collections in C# and some basic programs which will help beginners to quick start with Collections.
You may also be interested in...
- Slack Integration with C#
- Xamarin Forms: Getting Started
- Part 1: Introduction to C#
- Part 2: Arrays and Function in C#
- Part 3: OOPs Concepts in C#
- Part 4: Constructor/Destructor in C#
- Part 5: Abstract class in C#
- Part 6: Indexer,Delegates, Anonymous Method, Lambda expression in C#
- Part 7: Collections in C#
- Part 8: Generics in C#
- Part 9: Attributes in C#
- Part 10: Sealed/Partial class in C#
- Part 11: MultiThreading in C#
Happy Coding!
Discuss about post