Introduction to C# with basic programs

Introduction to C#:
This article is suited for beginners to intermediate programmers or anyone who would like to gain familiarity with the C# language. This article will help you get a quick head-start with C# language.
To get started, you will need a compiler and an editor. There are several options for obtaining a compiler to write C# programs. A free option is to download the .NET Frameworks SDK and use Notepad. Of course there are many editor and IDE options available, so see the Tools section to select the option that's right for you. Most of the examples in these tutorials run as console programs. Microsoft Visual Studio is also available in multiple versions as well as a free download for Visual Studio Express.
C# is a very powerful programming langauge by Microsoft. Its first version C# 1.0 released by Microsoft in June 2000 as part of its .NET framework. C# inherits many of the featurs of C, C++, Java and Visual Basic. In short C# is a general-purpose, type-safe, platform independent and robust object oriented programming language. The most current version of C#, as of this writting, is 7.3 which is released in 2018. C# has been derived from the C programming language and it has many features similar to C programming language. Similar to Java, it is object-oriented and comes with an extensive class library, supports exception handling, multiple types of polymorphism & interfaces.
Important:
This article assumes that you have installed Microsoft Visual Studio 2012 or later. Infact Visual Studio 2012, like its predecessor versions of Visual Studio, is a tool-rich development environment, providing you every tool that you may need to develop small or large scale C# projects.
Data Types In C#:
C# is a strongly typed language. It means, that you cannot use variable without data types. Data types tell the compiler that which type of data is used for processing. C# provides two types of data types: Value types and Reference types.
A Value type data type stores copy of the value whereas the Reference type data types stores the address of the value. C sharp provides great range of predefined data types but it also gives the way to create user defined data types.
Value Type Data Types:
Data Types | Size | Values |
---|---|---|
sbyte | 1 byte | -128 to 127 |
byte | 1 byte | 0 to 255 |
short | 1 byte | -32,768 to 32,767 |
ushort | 2 byte | 0 to 65,535 |
int | 4 byte | -2,147,483,648 to 2,147,483,647 |
uint | 4 byte | 0 to 4,294,967,295 |
long | 8 byte | -9223372036854775808 to 9223372036854775807 |
ulong | 8 byte | 0 to 18,446,744,073,709,551,615 |
char | 2 byte | 0 to 65535 |
float | 4 byte | -1.5 x 1045 to 3.4 x 1038 |
double | 8 byte | -5 x 10324 to 1.7 x 10308 |
decimal | 12 byte | -1028 to 7.9 x 1028 |
bool | --- | True or false |
Reference Type Data Types:
Data Types | Size | Values |
---|---|---|
string | Variable length | 0-2 billion Unicode characters |
object | --- | --- |
Note:
In C Language, char datatype uses ASCII code. ASCII values will be up to 255. ASCII will use 1 byte for 1 character. In C#, char and string datatype will uses UNICODE. UNICODE values will be up to 65535. UNICODE supports English and other popular languages like French, Latin, Greek etc. UNICODE will use 2 bytes for 1 character.
Difference between Value Type Datatypes and Reference Type Datatypes:
Value Types | Reference Types | |
---|---|---|
1 | The Structures are considered as value type datatypes. | The classes are considered as Reference type datatypes. |
2 | Value Type datatypes will use stack memory. | Reference Type datatypes will use heap memory. |
3 | Stack memory can be called as a static or compile time memory. | Heap memory can be called as a dynamic or run time memory. |
4 | Stack memory will store values. | Heap memory will store reference or memory address or memory location of the value. |
5 | Stack memory is used to store small amount of data. | Heap memory is used to store large amount of data. |
6 | Value type datatypes required initial or default value. | Reference type datatypes need not require initial or default value. |
7 | Garbage collection will not work with stack memory. | Garbage collection will work with heap memory. |
Important:
Object class is the super class in .Net. The datatypes and remaining classes are derived or created from Object class. It is in a System namespace . Namespace is collection of a classes and interfaces. In namespace hierarchy first namespace is System.
Operators in C#:
Operators are used for building expressions in C#. To calculate the value of variable or perform operation in variable you will have to make proper expression. These expressions are made using C# operators.
Operators are very useful thing in computing value. While writing a program, you need various types of operators to calculate value. These operators are categorized as different categories in C sharp tutorial that performs specific task. C# provides wide range of operators as follows:
- Arithmetic Operators: +, -, /, *, %
- Relational Operators: <, >, <=, >=, !=
- Logical Operators: &&, ||, !
- Comparison Operator: ==
- Concatenation Operator: +
- Assignment Operator: =
- Increment/Decrement Operators: ++, --
- Conditional Operators: ?:
- Index Operator: []
- Delegate Operator: +=, -=
- Member access Operator: .
- Memory allocation operator: new
Escape Sequences in C#:
- \n: New line
- \t: Tab space
- \v: Vertical tab
- \r: Return
- \a: Bell or beep
- \0: Null
Lets start with programming:
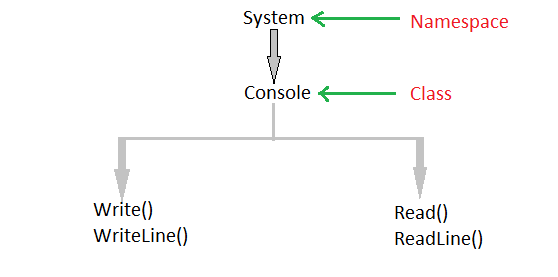
Write() is used to display text in console window. WriteLine() is used to display text or result in console window and after that cursor will go to next line. Read() is used to read one character from console window. ReadLine() is used to read set of characters from console window.
Structure of C# Program:
C# programs contains following sections:
- Using
- Namespace
- Class
- Main()
Using is called directive. It is used to import predefined namespaces in c# application. In C# application file name will be used as a namespace name. By default it contains one class. Static is used to preserve memory for the method, so that it can be stored and will be executed. Void is an empty datatype which does not returns anything. Main() is the entry point for the program. Program execution starts with a main(). In C#, main() contains one argument. If c# program is executed at command prompt then to send the values to the program then command line argument is used.
Programs:
1. Hello World:
using System; namespace HelloWorld { class Program { static void Main(string[] args) { Console.WriteLine("Hello World"); Console.ReadLine(); } } }
Output:
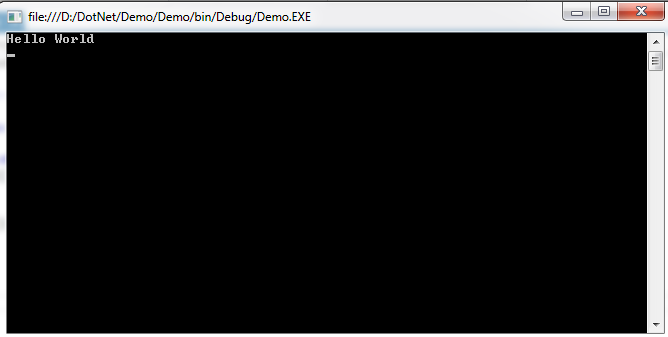
2. Accept name from user and display it:
using System; namespace HelloWorld { class Program { static void Main(string[] args) { string s; Console.WriteLine("Enter Name:"); s = Console.ReadLine(); Console.WriteLine("Name is: " + s); Console.Read(); } } }
Output:
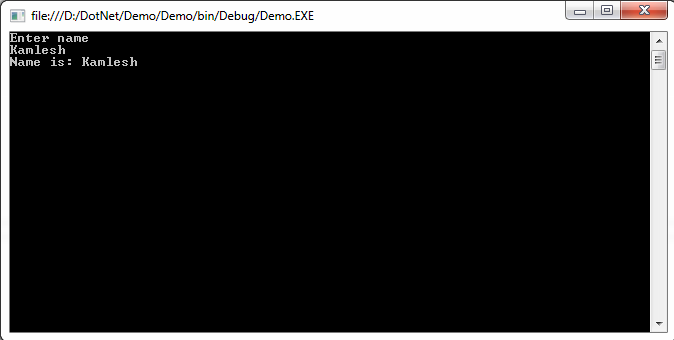
Note:
C# contains two types of notations. They are pascal notation and camel notation. In C# namespace, class and method names will be in pascal notations(Title case). And variable names will be in camel notation. Console class in System namespace contains static methods. They can be accessed with the class name. Dot(.) is called member access operator. It is used to access variables and methods in the class. In C# the line should be terminated with ';'. To execute the program, press F5 or click on start debugging icon in the tool bar.
3. Print sum of two numbers:
using System; namespace Addition { class Program { static void Main(string[] args) { int a, b; Console.WriteLine("Enter Values:"); a = int.Parse(Console.ReadLine()); b = int.Parse(Console.ReadLine()); Console.WriteLine("Sum is: " + a+b); Console.Read(); } } }
Output:
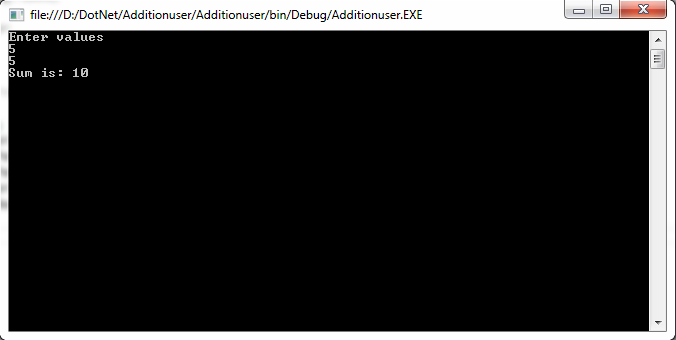
Note:
ReadLine() in a Console class will read any data in the string format.
int.Parse():- It is a predefined structure contains Parse(). It is used to convert string type data to int.
convert.ToInt32:- Convert class contains ToInt32 method. It is used to convert string to int.
4. Check Even or Odd Number:
using System; namespace EvenOdd { class Program { static void Main(string[] args) { int a; Console.WriteLine("Enter Value:"); a = int.Parse(Console.ReadLine()); if (a % 2 == 0) Console.WriteLine("Even Number"); else Console.WriteLine("Odd Number"); Console.Read(); } } }
Output:
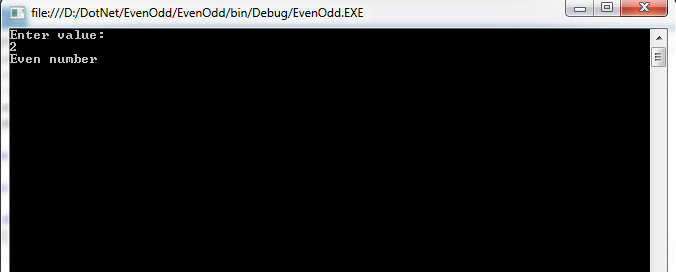
5. Accept three values and print biggest value:
using System; namespace LargestValue { class Program { static void Main(string[] args) { int a, b, c; Console.WriteLine("Enter Values:"); a = int.Parse(Console.ReadLine()); b = int.Parse(Console.ReadLine()); c = int.Parse(Console.ReadLine()); if (a > b && a > c) Console.WriteLine("Biggest Value : " +a); else if(b>c) Console.WriteLine("Biggest Value : " + b); else Console.WriteLine("Biggest Value : " + c); Console.Read(); } } }
Output:
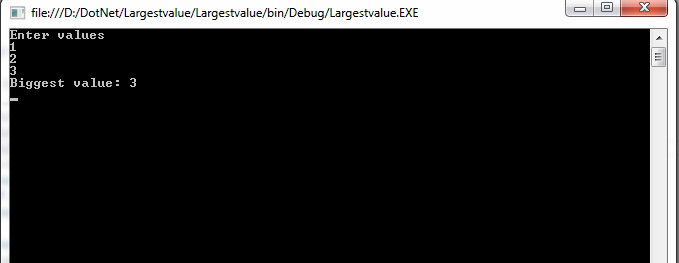
6. Accept digit and print it in character:
using System; namespace PrintCharacter { class Program { static void Main(string[] args) { int a; Console.WriteLine("Enter Digit:"); a = int.Parse(Console.ReadLine()); switch (a) { case 0: Console.WriteLine("Zero"); break; case 1: Console.WriteLine("One"); break; case 2: Console.WriteLine("Two"); break; default: Console.WriteLine("Invalid"); break; } Console.Read(); } } }
Output:
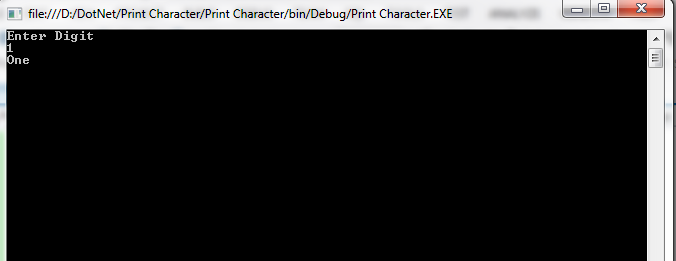
7. Display Table of given number:
using System; namespace Table { class Program { static void Main(string[] args) { int a; Console.WriteLine("Enter value:"); a = int.Parse(Console.ReadLine()); for (int i = 1; i <= 10; i++) { Console.WriteLine(a + "*" + i + "=" + a * i); } Console.Read(); } } }
Output:
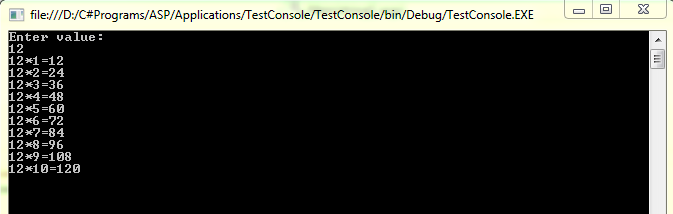
8. Print numbers using do_while loop:
using System; namespace DoWhile { class Program { static void Main(string[] args) { int a=1; do { Console.WriteLine(a); a = a + 1; } while (a <= 10); Console.Read(); } } }
Output:
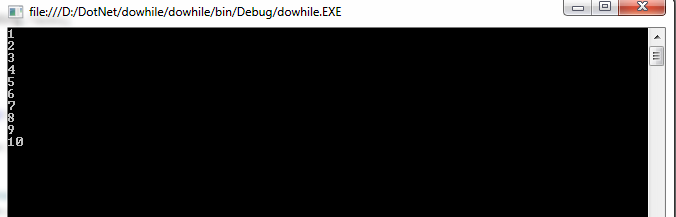
Boxing and Unboxing:
Boxing means converting value type to reference type. UnBoxing means converting reference type to value type.
9. Example of Boxing & Unboxing:
using System; namespace Boxing_Unboxing { class Program { static void Main(string[] args) { int a=123; object s; s = a; Console.WriteLine(s); int b; b = Convert.ToInt32(s); Console.WriteLine(b); Console.Read(); } } }
Output:
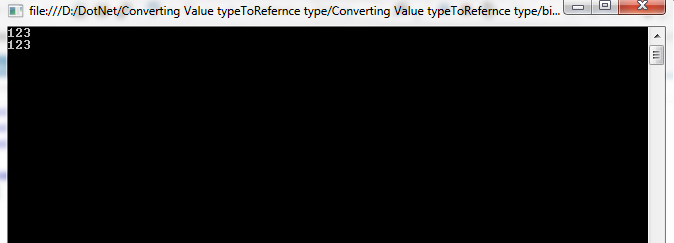
Note:
int datatype is structure. It is a value type datatype and will use the stack memory. Object datatype is a class. It is reference type datatype. It will use heap memory. s=a means the reference or memory address of the value will be store in s.
b=Convert.ToInt32(s): The value in the memory address of reference in s will be stored in variable b.
C# contains two types of comments:
- Single line comment: //
- Multi Line comment: /*.......*/
In this way, we learned introduction to C# and some basic programs which will help you to quick start with your journey in to C# world.
You may also be interested in...
- Slack Integration with C#
- Xamarin Forms: Getting Started
- Part 1: Introduction to C#
- Part 2: Arrays and Function in C#
- Part 3: OOPs Concepts in C#
- Part 4: Constructor/Destructor in C#
- Part 5: Abstract class in C#
- Part 6: Indexer,Delegates, Anonymous Method, Lambda expression in C#
- Part 7: Collections in C#
- Part 8: Generics in C#
- Part 9: Attributes in C#
- Part 10: Sealed/Partial class in C#
- Part 11: MultiThreading in C#
Happy Coding!
Discuss about post