Generics in C#

What is Generics?
Generic is also a class used to store similar values according to the specified datatype. Generics are called as type safe. According to the given datatype only the similar data will be stored. The predefined generics are existing in System.Collections.Generic namespace. Generics in C# are similar to templates in C++.
1. List:
It is used to store similar values according to the specified datatypes.
Examples:
1. Example of List:
using System; using System.Collections; using System.Collections.Generic; namespace Demo11 { class Demo { public static void Main(string[] args) { List<int> obj1 = new List<int>(); obj1.Add(5); obj1.Add(10); obj1.Add(15); { foreach (int a in obj1) { Console.WriteLine(a); } List<string> obj2 = new List<String>(); obj2.Add("abc"); obj2.Add("pqr"); obj2.Add("xyz"); foreach (String b in obj2) { Console.WriteLine(b); } List<double> obj3 = new List<double>(); obj3.Add(4.5); obj3.Add(5.6); obj3.Add(6.5); foreach (double c in obj3) { Console.WriteLine(c); } Console.Read(); } } } }
Output:
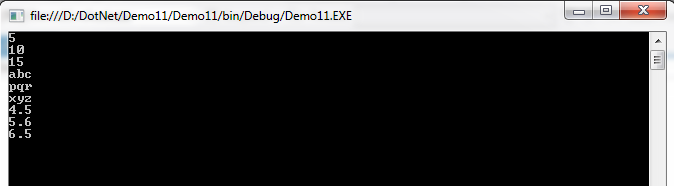
2. Example of List using Property:
using System; using System.Collections; using System.Collections.Generic; namespace Demo11 { class Customer { private int cid; private String cname; public Customer(int cid, string cname) // Creating Constructor { pcid = cid; pcname = cname; } public int pcid // Property name { get { return cid; } set { cid = value; } } public String pcname { get { return cname; } set { cname = value; } } } class Demo { public static void Main(string[] args) { List<Customer> obj = new List<Customer>(); obj.Add(new Customer(101, "Kamlesh")); //Calling Constructor obj.Add(new Customer(102, "Dotnetwisdom")); obj.Add(new Customer(103, "csharpcorner")); foreach (Customer c in obj) { Console.WriteLine(c.pcid + " " + c.pcname); } Console.Read(); } } }
Output:
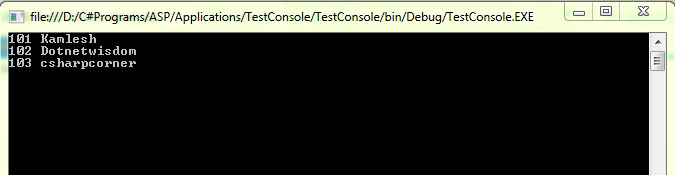
2. Dictionary:
It is used to store values in Key/value pair. For key/value datatype should be given.
Example of Dictionary:
using System; using System.Collections; using System.Collections.Generic; namespace Demo11 { class Demo { public static void Main(string[] args) { Dictionary<int, String> obj = new Dictionary<int, String>(); obj.Add(1, "Hi"); //Calling Constructor obj.Add(2, "How are you"); obj.Add(3, "Have a nice day"); obj.Add(4, "By"); foreach ( var s in obj) { Console.WriteLine(s.Key + " " + s.Value); } Console.Read(); } } }
Output:
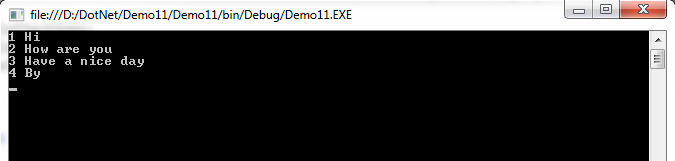
Note:
var is a predefined structure used to work with any type of data. It is used to access values from Dictionary generic. It contains key/value property. By using them key/value can be accessed from generic.
In this way, we learned Generics in C# and some basic programs which will help beginners to quick start with Generics.
You may also be interested in...
- Slack Integration with C#
- Xamarin Forms: Getting Started
- Part 1: Introduction to C#
- Part 2: Arrays and Function in C#
- Part 3: OOPs Concepts in C#
- Part 4: Constructor/Destructor in C#
- Part 5: Abstract class in C#
- Part 6: Indexer,Delegates, Anonymous Method, Lambda expression in C#
- Part 7: Collections in C#
- Part 8: Generics in C#
- Part 9: Attributes in C#
- Part 10: Sealed/Partial class in C#
- Part 11: MultiThreading in C#
Happy Coding!
Discuss about post