Abstract class in C#

Introduction:
It is similar to a class but instance or object cannot be created with abstract class. Abstract class contains variables, methods & abstract methods. And the abstract methods in abstract class will be created in derived class, so abstract method will be always used as a base class.
Features Of Abstract class:
- An abstract class can have constructors or destructors.
- An abstract class cannot support multiple inheritance.
- An abstract class can inherit from a class and one or more interfaces.
- An abstract class can implement code with non-Abstract methods.
- An Abstract class can have constants and fields.
Examples:
1) Abstract class containing method & abstract method:
using System; namespace Demo11 { abstract class clsEmp { public void getEmpDetails(int eno, string ename) { Console.WriteLine(eno+" "+ename); } public abstract void getDeptDetails(int dno, string dname); } class clsDept : clsEmp { public override void getDeptDetails(int dno, string dname) { Console.WriteLine(dno + " " + dname); } } class Test { static void Main(string[] args) { clsDept obj = new clsDept(); obj.getEmpDetails(1, "Kamlesh"); obj.getDeptDetails(10, "Software"); Console.ReadLine(); } } }
Output:

Note:
When abstract method created in derived class then it should be created with override. So that it can override the abstract method in base class. Abstract class is used for data hiding. To hide the class, variables and methods in the class then abstract class is used.
2) Abstract class containing variables and methods:
using System; namespace Demo11 { abstract class clsEmp { public int eno; public string ename; public void showDetails() { Console.WriteLine(eno + " " + ename); } } class clsEmp1 : clsEmp { } class Test { static void Main(string[] args) { clsEmp1 obj = new clsEmp1(); obj.eno=111; obj.ename="Kamlesh"; obj.showDetails(); Console.ReadLine(); } } }
Output:

3) Abstract class containing only abstract method:
using System; namespace Demo11 { abstract class abc { public abstract void getstring(); } class xyz:abc { public override void getstring() { Console.WriteLine("Kamlesh Dot Net"); } } class Test { static void Main(string[] args) { xyz obj = new xyz(); obj.getstring(); Console.ReadLine(); } } }
Output:

Property or Smart field:
If the class contains private variables, to access those variables in main(), then property is used. It contains get and set blocks. Get is used to access the value and set is used to assign the value. Property can also be called as Smart Field.
4) Example of Property:
using System; namespace Demo11 { class clsInfo { private string s; private bool b; public string getString { get { return s; } set { s = value; } } public bool getBool { get { return b; } set { b = value; } } } class Test { static void Main(string[] args) { clsInfo obj = new clsInfo(); obj.getString = "Kamlesh"; obj.getBool = true; Console.WriteLine(obj.getString); Console.WriteLine(obj.getBool); Console.ReadLine(); } } }
Output:
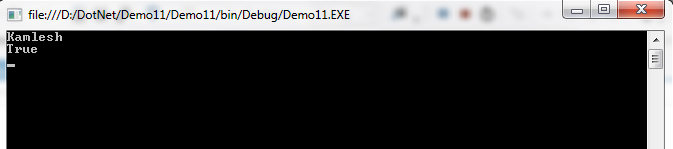
In this way, we have learned abstract class in C# with examples in this article. I hope this will help beginners to understand what is abstract class and how to implement it.
You may also be interested in...
- Slack Integration with C#
- Xamarin Forms: Getting Started
- Part 1: Introduction to C#
- Part 2: Arrays and Function in C#
- Part 3: OOPs Concepts in C#
- Part 4: Constructor/Destructor in C#
- Part 5: Abstract class in C#
- Part 6: Indexer,Delegates, Anonymous Method, Lambda expression in C#
- Part 7: Collections in C#
- Part 8: Generics in C#
- Part 9: Attributes in C#
- Part 10: Sealed/Partial class in C#
- Part 11: MultiThreading in C#
Happy Coding!
Discuss about post